Introduction
In CSS, a lot of the time when we want to give children elements under the same parent the same CSS property, we will use compound CSS selector like the following:
.container .title,
.container .detail {
color: black;
}
It allows us to select multiple elements at once and apply the same CSS property to them. However, as the list grows longer we will have to repeat the parent class multiple times. This is when the :is selector comes into play to solve this issue.
Example
Given the following HTML:
<div class="card --card1">
<h2 class="title">Normal CSS</h2>
<p class="detail">
.--card1 .title,
.--card1 .detail {
color: brown;
}
</p>
</div>
Suppose we want to mark both the title element and the detail class element to be brown, we will write the following CSS:
/* Normal CSS */
.--card1 .title,
.--card1 .detail {
color: brown;
}
And it will give us the following result:
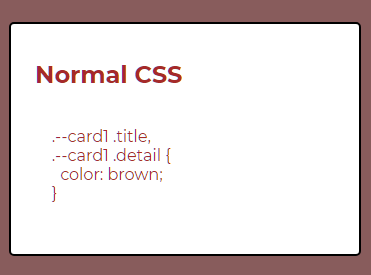
While the above CSS works, it’s kind of redundant to repeat the parent class multiple times. Instead, we can achieve the same effect with the :is selector.
/* :is selector */
.--card2 :is(.title, .detail) {
color: brown;
}
And the result will look the same:
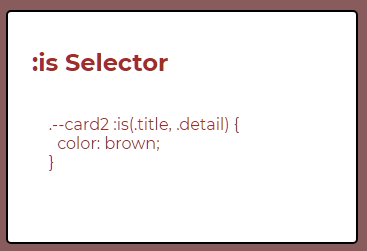
The CSS we wrote with :is selector basically means the same thing as if we repeat the parent element class twice, but allows us to write less code.
Common issue with CSS
A common issue that we run into no matter if you are using CSS or SCSS is that when any of the elements in your CSS selector has typo, the entire chunk of CSS selector will not work.
Suppose we have a typo in our CSS, so instead of .detail we wrote :detail.
/* Normal CSS with errors */
.--card3 .title,
.--card3 :detail {
color: brown;
}
This will produce the following outcome:
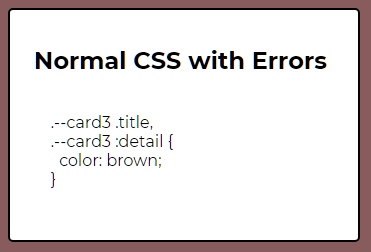
Not only did the detail element not get the CSS property we want, the title element also didn’t get the CSS property properly. This is because browsers interpret the entire chunks of CSS together, so when one element has typos, the entire CSS selector will be ignored.
When the similar situation happens with the :is selector, a different result will happen.
/* :is selector with errors */
.--card4 :is(.title, :detail) {
color: brown;
}
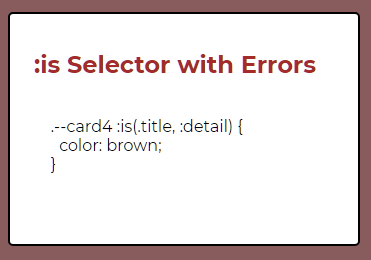
As you can see, even though we have a typo when we try to reference the detail element, the title element can still get the CSS property as expected. The :is selector is more forgiving.
The source code of this tutorial can be found on the following CodePen:
https://codepen.io/chen1223/pen/vYyzzLN
Be sure to stop by the CodePen and to play around with the four cards we described in this tutorial.
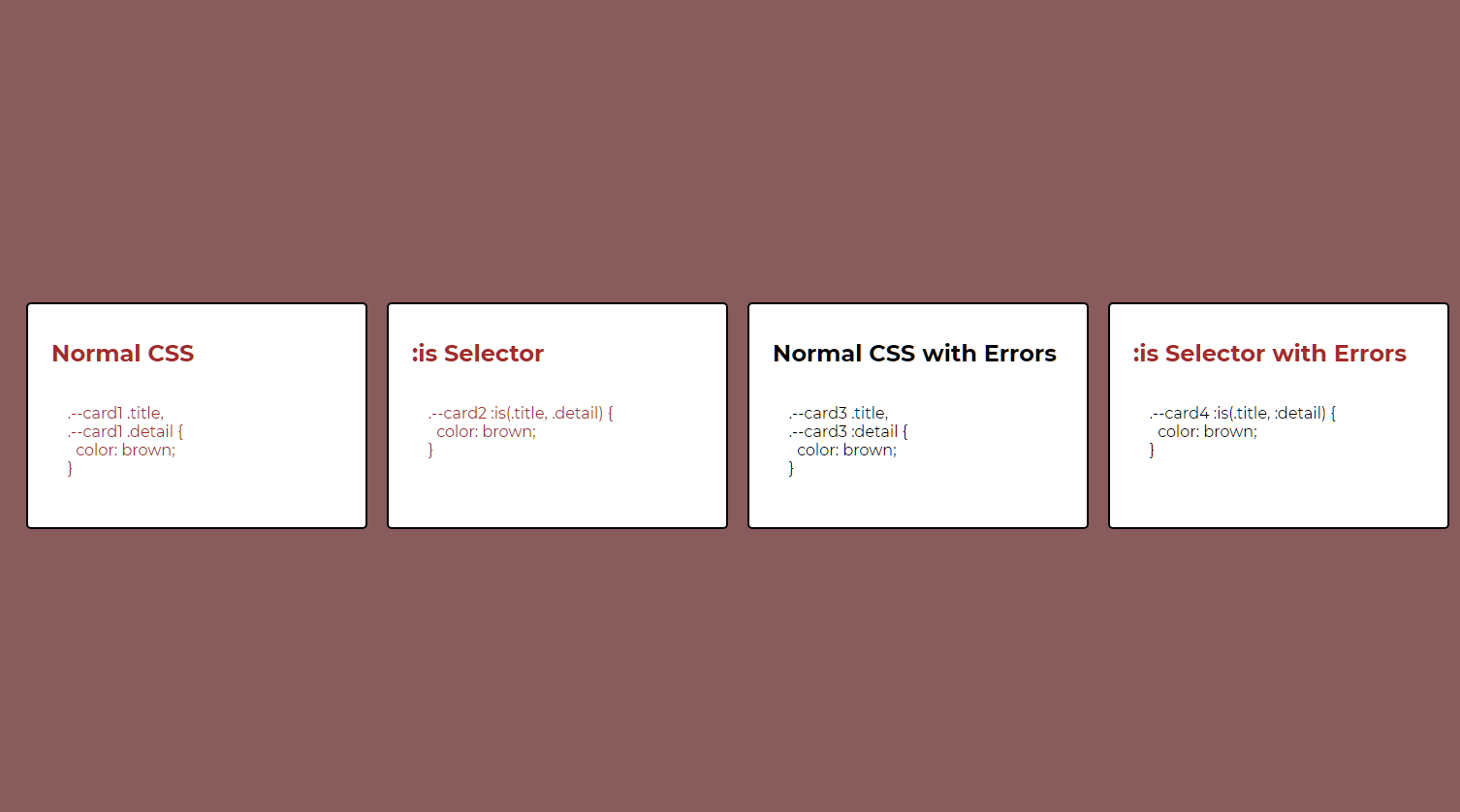
Browser support
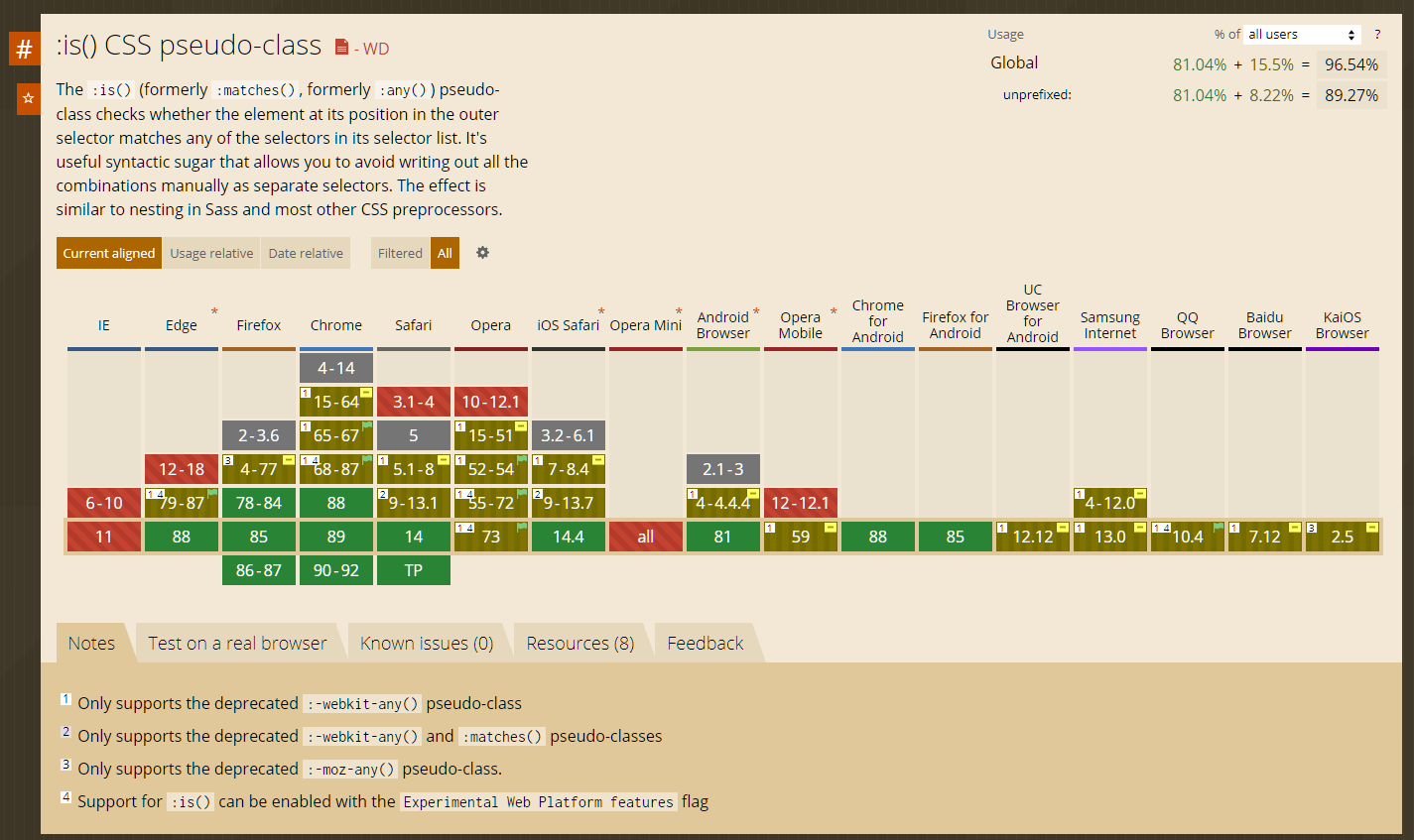
In terms of browser support, all major browsers except Internet Explorer and Opera Mini have good support on the :is selector. So start using the :is selector and make your CSS code more elegant.